পোস্ট এ: জুল 14, 2024 - 104 ভিউ
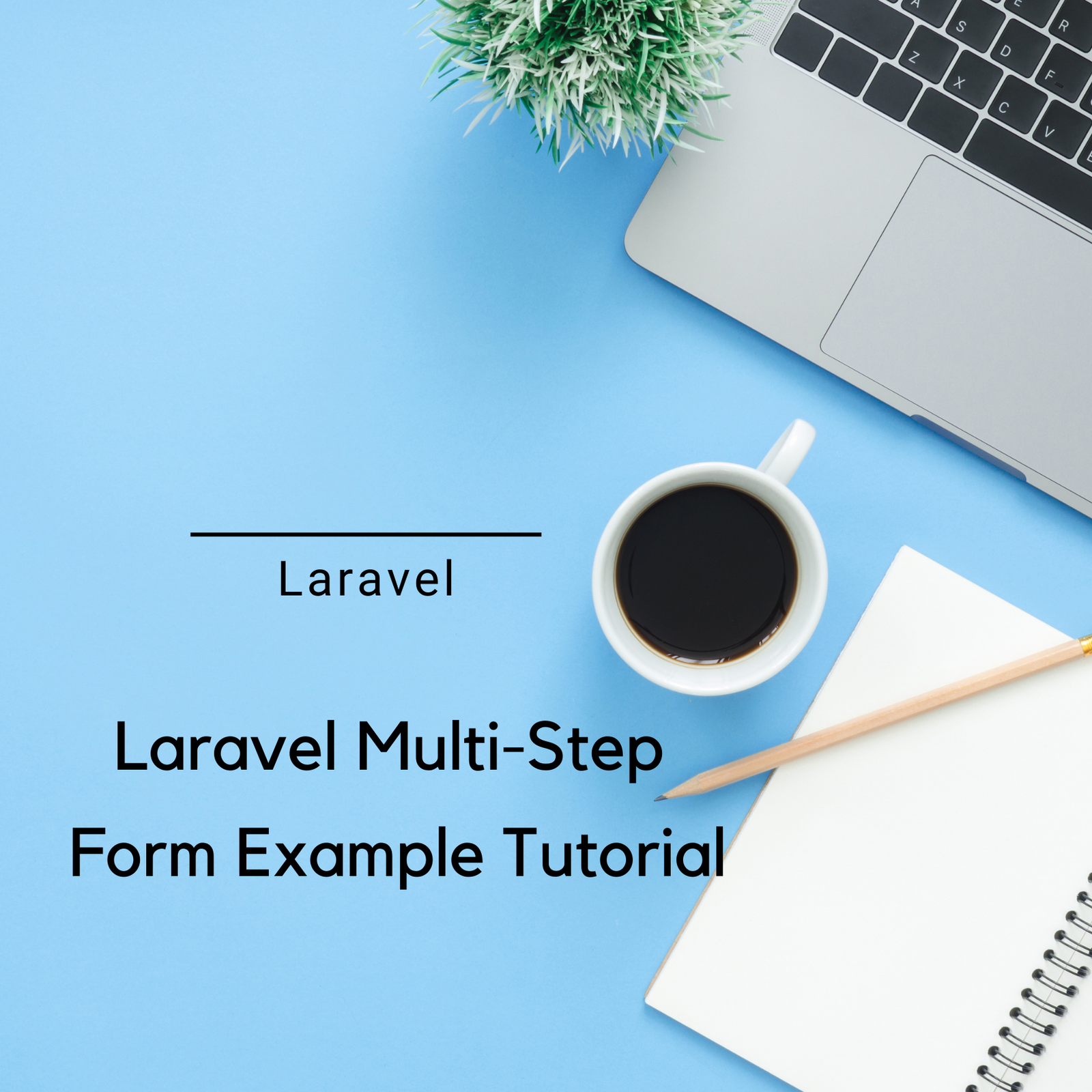
Laravel Multi-Step Form Example Tutorial
In this tutorial, we'll walk through the process of creating a multi-step form in Laravel. This will include setting up a new Laravel project, creating the form, handling form data, and validating user input across multiple steps.
Prerequisites
- PHP installed on your system
- Composer globally installed
- Basic understanding of Laravel
Step 1: Setting Up a New Laravel Project
First, let's create a new Laravel project. Open your terminal and run:
composer create-project --prefer-dist laravel/laravel laravelMultiStepForm
Navigate to your project directory:
cd laravelMultiStepForm
Step 2: Setting Up the Database
Edit the .env
file to configure your database settings. Here's an example using MySQL:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel_form
DB_USERNAME=root
DB_PASSWORD=
Run the migration to create the database tables:
php artisan migrate
Step 3: Creating the Multi-Step Form
Create the Controller
Generate a new controller for handling the form logic:
php artisan make:controller FormController
Inside FormController
, add methods for each step of the form. For example:
public function showFormStepOne(Request $request)
{
return view('form-step-one');
}
public function postFormStepOne(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|max:255',
'email' => 'required|email',
]);
$request->session()->put('stepOne', $validatedData);
return redirect()->route('form.step.two');
}
Repeat for additional steps.
Create the Views
Create views for each form step. Here's an example for form-step-one.blade.php
:
<form method="POST" action="{{ route('form.step.one.post') }}">
@csrf
<label for="name">Name:</label>
<input type="text" name="name" required>
<label for="email">Email:</label>
<input type="email" name="email" required>
<button type="submit">Next</button>
</form>
Create similar views for other steps.
Step 4: Managing Routes
Add routes for each step in routes/web.php
:
Route::get('/form-step-one', 'FormController@showFormStepOne')->name('form.step.one');
Route::post('/form-step-one', 'FormController@postFormStepOne')->name('form.step.one.post');
Add similar routes for other steps.
Step 5: Handling Session Data
Ensure that data from previous steps is stored in the session and is available in subsequent steps. Validate and redirect as necessary.
Step 6: Completing the Form
Create a final method in FormController
to handle the submission of the complete form:
public function completeForm(Request $request)
{
$allData = $request->session()->all();
// Process or store the data
$request->session()->forget(['stepOne', 'stepTwo']); // etc.
return view('form-complete', compact('allData'));
}